5 Advanced Python Features for Faster Coding
Accelerate your Python development with these five powerful features—list comprehensions, lambda functions, decorators, and more—plus bite-sized CodenQuest exercises to sharpen your skills.
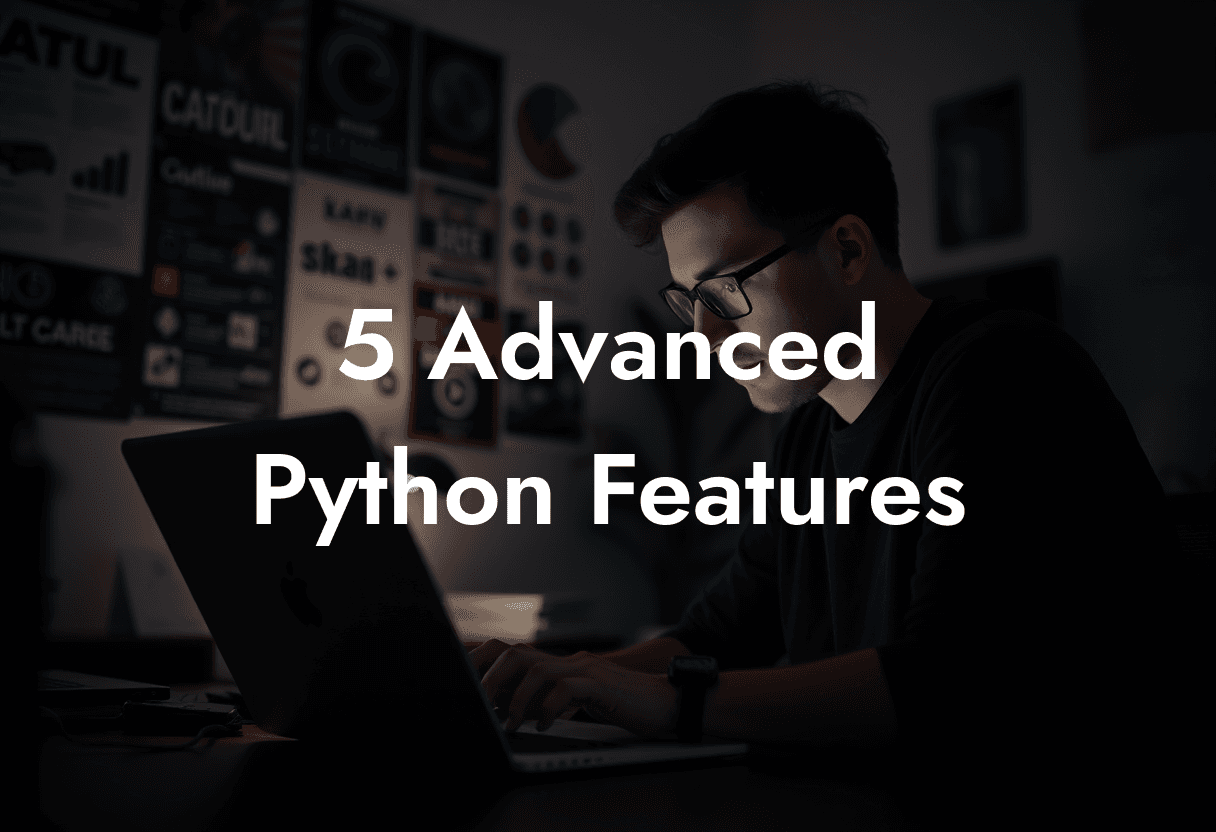
If you’ve spent any time working with Python, you already know the language is celebrated for its readability and efficiency. But there’s more than just the basics—Python offers powerful “advanced” features that can significantly speed up your workflow and reduce boilerplate code. In this post, we’ll explore five such features, with examples and bite-sized challenges you can practice right away on CodenQuest. By the end, you’ll have new tools in your arsenal for writing cleaner, more concise Python code at lightning speed.
1. List Comprehensions
Why They’re Useful
List comprehensions allow you to build new lists in a single line of code, often replacing multi-line loops and conditional statements. They are not only more concise but also typically more readable, making your code easier to understand at a glance.
Basic Syntax
# Traditional approach
numbers = [1, 2, 3, 4, 5]
squares = []
for num in numbers:
squares.append(num ** 2)
# Using list comprehensions
numbers = [1, 2, 3, 4, 5]
squares = [num ** 2 for num in numbers]
In the second approach, you accomplish the same task with fewer lines and a cleaner structure.
Conditional List Comprehensions
You can also include if-conditions to filter out certain values.
# Keep only even numbers
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [num for num in numbers if num % 2 == 0]
print(even_numbers) # [2, 4, 6]
2. Lambda Functions
Why They’re Useful
Lambda functions are anonymous functions—one-line pieces of code that can be particularly handy for simple, “throwaway” operations. They’re often used in conjunction with built-in functions like map
, filter
, and sorted
.
Basic Syntax
# General syntax
lambda arguments: expression
# Example
multiply_by_two = lambda x: x * 2
print(multiply_by_two(5)) # 10
Using Lambdas with Map & Filter
numbers = [1, 2, 3, 4, 5]
doubled = list(map(lambda x: x * 2, numbers))
print(doubled) # [2, 4, 6, 8, 10]
evens = list(filter(lambda x: x % 2 == 0, numbers))
print(evens) # [2, 4]
While lambdas can be powerful, be cautious about readability—if a function is more than a few lines, it’s typically better to define it with def.
Mini Exercise on CodenQuest
Prompt: “Use a lambda function to filter out names from a given list that start with the letter 'A'.”
names = ["Alice", "Bob", "Amy", "George", "Anne"]
a_names = list(filter(lambda name: name.startswith('A'), names))
print(a_names) # ['Alice', 'Amy', 'Anne']
3. Decorators
Why They’re Useful
Decorators allow you to modify or enhance the behavior of functions (or classes) without changing their source code. You “wrap” your function with another function, which can inject additional functionality.
Basic Decorator Example
def my_decorator(func):
def wrapper():
print("Before function call")
func()
print("After function call")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
# Output:
# Before function call
# Hello!
# After function call
In this example, @my_decorator
is syntactic sugar for say_hello = my_decorator(say_hello)
.
Use Cases
- Logging: Automatically log every time a function runs.
- Access Control: Check user permissions before allowing a function to execute.
- Caching: Store function outputs to speed up repeated calculations.
Mini Exercise on CodenQuest
Prompt: “Create a decorator that times how long a function takes to execute and prints out the duration.”
import time
def timer_decorator(func):
def wrapper(*args, **kwargs):
start = time.time()
result = func(*args, **kwargs)
end = time.time()
print(f"Execution time: {end - start} seconds.")
return result
return wrapper
@timer_decorator
def sum_of_n(n):
return sum(range(1, n + 1))
print(sum_of_n(1000000))
4. Generator Expressions
Why They’re Useful
Generators are similar to list comprehensions but don’t construct the entire list in memory right away. Instead, they yield items one at a time, making them more memory-efficient for large data sets.
Basic Syntax
numbers = (num * num for num in range(1, 1000000))
# This creates a generator object instead of a list
# Access items
for _ in range(5):
print(next(numbers))
# Outputs 1, 4, 9, 16, 25 (first 5 squares)
Generator expressions use parentheses instead of square brackets, which tells Python to create a generator rather than a list.
Generator Functions
You can also define a generator with the yield
keyword inside a function:
def fibonacci(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
fib_sequence = fibonacci(10)
print(list(fib_sequence)) # [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
5. Context Managers
Why They’re Useful
Context managers handle setup and teardown logic automatically. You’ve likely seen this with Python’s with
statement for file operations, ensuring files are safely closed after use—even if an exception occurs.
Basic Syntax with with
with open("data.txt", "r") as file:
content = file.read()
print(content)
Under the hood, this ensures file.close()
is called when you exit the with
block.
Creating Your Own Context Manager
You can create custom context managers using classes with __enter__
and __exit__
methods, or by using the contextlib
module.
class FileOpener:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
def __enter__(self):
self.file = open(self.filename, self.mode)
return self.file
def __exit__(self, exc_type, exc_value, traceback):
self.file.close()
with FileOpener("example.txt", "w") as f:
f.write("Hello, World!")
Mini Exercise on CodenQuest
Prompt: “Create a custom context manager that measures and prints the time spent inside a with
block.”
import time
class Timer:
def __enter__(self):
self.start = time.time()
return self
def __exit__(self, exc_type, exc_value, traceback):
end = time.time()
print(f"Duration: {end - self.start} seconds.")
with Timer():
# Some code you want to time
total = 0
for i in range(1000000):
total += i
6. Practice These Features on CodenQuest
By now, you’ve seen how list comprehensions, lambda functions, decorators, generator expressions, and context managers can supercharge your Python coding. But reading about them is one thing—mastery comes from practice.
CodenQuest: Your Practice Hub
- Bite-Sized Exercises: Short problems focusing on each advanced feature, similar to Mimo’s style. Perfect for quick learning sessions.
- Immediate Feedback: Get test case results in real time and tweak your code on the spot.
- Gamification: Earn experience points, keep up your coding streak, and challenge others in the community.
- Premium Perks:
- Unlimited Lives – No interruptions in your coding flow.
- AI Coding Assistant – Get real-time suggestions or debugging tips as you tackle advanced Python challenges.
7. Conclusion
Knowing these five advanced Python features can transform the way you code:
- List Comprehensions let you handle data transformations in just one line.
- Lambda Functions give you quick, anonymous operations for map, filter, and more.
- Decorators wrap functions with extra functionalities like logging or timing.
- Generator Expressions provide memory-efficient iterations.
- Context Managers simplify setup and teardown tasks for safer, more readable code.
Each of these techniques offers speed, clarity, or both—helping you write more polished, professional-grade Python applications. Don’t forget to solidify your new knowledge by practicing on CodenQuest. The more you use these features, the more natural they’ll become, saving you time in your day-to-day coding tasks.
Additional Resources
- External Links:
Thank you for reading! Continue exploring these tools, challenge yourself on daily exercises, and watch your Python skills skyrocket. If you’re looking for structured, gamified practice that keeps you motivated, be sure to explore the advanced tracks on CodenQuest—because fast and efficient coding is just a few features away.