Getting Started with Python: A Comprehensive Beginner’s Guide with CodenQuest
Learn the fundamentals of Python—syntax, variables, and data types—and discover how CodenQuest can help you master coding exercises efficiently. Follow this guide to get started on your Python journey!
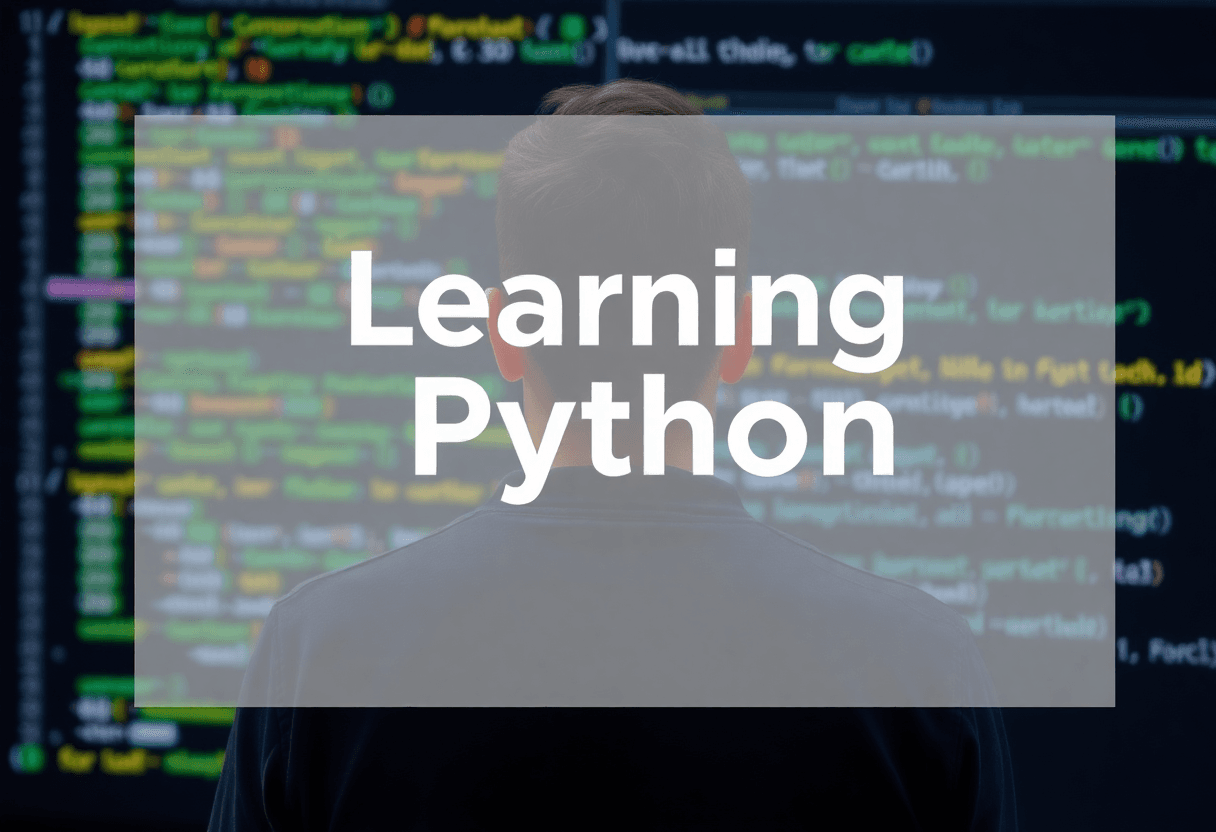
Getting Started with Python: A Comprehensive Beginner’s Guide with CodenQuest
Whether you’re just dipping your toe into programming or aiming to boost your coding skills to land your dream job, Python is one of the most beginner-friendly and powerful languages you can learn. Known for its readable syntax and massive community support, Python powers everything from web development to data science and artificial intelligence. In this article, we’ll discuss the essential building blocks of Python—covering its syntax, variables, and data types—and show you how to practice them efficiently using CodenQuest, our gamified coding platform available on iOS, Android, and the web.
1. Why Learn Python?
Python is consistently ranked as one of the most popular programming languages in the world—and for good reason:
- Readability & Simplicity: Python’s syntax is designed to be clean and intuitive. It uses fewer lines of code to accomplish tasks compared to languages like Java or C++.
- Versatility: From web development frameworks like Django and Flask, to data science libraries like NumPy, pandas, and scikit-learn, Python has a vast ecosystem of tools.
- High Demand: Developers with Python expertise are sought after in many industries—be it tech startups, major corporations, or research labs.
If you’re just getting started or aiming to sharpen your skills, Python is an excellent choice. And with platforms like CodenQuest, you can learn and practice Python in a fun, engaging manner, complete with gamified mechanics such as streaks, leaderboards, and daily challenges.
2. Setting Up Python on Your Machine
Before we dive into the basics, you might want to have Python installed locally. However, if you’re using CodenQuest, you can skip traditional setup and practice directly in your browser or on mobile without worrying about local environment configurations.
Installing Python Locally
- Download Python: Visit the official Python Downloads page to grab the latest version of Python (3.x).
- Run the Installer:
- On Windows, make sure to check “Add Python to PATH” during the installation process.
- On macOS, you can use Homebrew by typing
brew install python
in the Terminal.
- Verify Installation: Open your terminal or command prompt, type:If you see Python 3.x, you’re set.
python --version
Alternatively, you can skip all this and head straight to CodenQuest where Python code can be executed and tested within the app or in a web browser—no setup required!
3. Python Syntax: An Overview
Python’s syntax is both straightforward and flexible. Let’s jump right in with the classic “Hello, World!” example.
# Hello World in Python
print("Hello, World!")
- No semicolons: Unlike languages such as JavaScript or C++, Python does not require semicolons at the end of each statement.
- Indentation: Python uses indentation (spaces or tabs) to determine code blocks. For instance, inside functions or loops, you’ll add a level of indentation to denote scope.
Indentation Example
def greet(name):
# The code inside this function is indented
print(f"Hello, {name}!")
greet("Alice")
Here, the print
statement is indented to show that it belongs to the greet
function.
Comments
- Single-line comments start with
#
. - Multi-line comments often use triple quotes
""" ... """
, although that’s more of a docstring convention in Python.
# This is a single-line comment
"""
This is a multi-line comment
or docstring, which can
span multiple lines.
"""
Pro Tip: Keep your code clean and use comments sparingly—let your code speak for itself whenever possible.
4. Working with Variables in Python
Variables in Python don’t require an explicit type declaration. Simply assign a value, and Python determines the variable type at runtime.
Variable Declaration
message = "Hello, CodenQuest!" # String variable
age = 25 # Integer variable
height = 5.9 # Float variable
is_student = True # Boolean variable
- You can reassign variables with different data types as needed. For example:
x = 10 # x is an integer
x = "Ten" # Now, x is a string
- While this flexibility is useful, you should keep track of how your variables are used to avoid confusion in larger projects.
Naming Conventions
- Snake Case: By convention, Python variable names are written in lower case using underscores to separate words (e.g.,
my_variable
). - Descriptive Names: Use clear, descriptive variable names so anyone reading your code understands its purpose.
Check out Python Problems on CodenQuest
5. Data Types in Python
Python comes with several built-in data types. Understanding these is vital for writing clean, efficient code.
-
Numeric Types:
- int (e.g.,
42
) - float (e.g.,
3.14
) - complex (e.g.,
2+3j
– less common for beginners)
- int (e.g.,
-
String:
- Defined with single or double quotes:
"Hello"
or'Hello'
. - Supports slicing and built-in methods like
upper()
,lower()
,split()
, etc.
text = "Python" print(text[1]) # y print(text.upper()) # PYTHON
- Defined with single or double quotes:
-
Boolean:
True
orFalse
.- Often used in conditionals (
if
,while
, etc.).
-
List:
- Ordered, mutable collections of items.
- Defined in square brackets
[ ]
.
fruits = ["apple", "banana", "cherry"] fruits.append("orange") print(fruits) # ['apple', 'banana', 'cherry', 'orange']
-
Tuple:
- Ordered but immutable sequences.
- Defined in parentheses
( )
.
coordinates = (10, 20) print(coordinates[0]) # 10 # coordinates[0] = 30 # Error: tuples are immutable
-
Dictionary:
- Key-value pairs, defined with curly braces
{ }
.
person = { "name": "Alice", "age": 28 } print(person["name"]) # Alice
- Key-value pairs, defined with curly braces
-
Set:
- Unordered collections of unique items.
- Useful for membership tests or removing duplicates.
unique_numbers = {1, 2, 2, 3, 4} print(unique_numbers) # {1, 2, 3, 4} (duplicates removed)
Code Sample: Data Types in Action
# Working with multiple data types
name = "Bob"
age = 30
hobbies = ["chess", "coding", "music"]
info = {
"occupation": "Data Analyst",
"city": "New York"
}
print(f"Name: {name}, Age: {age}")
print(f"First Hobby: {hobbies[0]}")
print(f"Occupation: {info['occupation']}")
Note: Once you understand Python’s data types, you can start building more complex structures and algorithms. When you’re ready to practice, head over to CodenQuest’s dedicated Python Practice Section.
6. Practicing Python on CodenQuest
CodenQuest is a gamified platform designed for learners and professionals who want to improve their coding skills in a fun and interactive way. Here’s how you can make the most of CodenQuest when learning Python:
- Bite-Sized Lessons: For absolute beginners, our Python track offers short, digestible lessons that guide you through variables, data types, and syntax step by step—similar to Mimo’s approach.
- Code Editor with Auto-Completion: CodenQuest’s integrated code editor helps speed up your practice by suggesting possible completions and syntax prompts.
- Immediate Feedback: Run your code directly in the browser or mobile app and get results instantly. If your solution needs improvement, test cases will provide guidance on what went wrong.
- Gamification Elements:
- Daily Challenges: Receive small, bite-sized tasks every day to maintain your coding streak.
- Weekly League: Compete with friends and other learners, earning points for solving problems quickly and efficiently.
- Statistics & Code Performance: Track how long you took, how many attempts you made, and how your solution stacks up in terms of efficiency.
Sample Exercise: Print a Personalized Greeting
For a beginner-level exercise on CodenQuest, you might see a prompt like:
> Prompt: Write a function greet_user(name) that prints "Hello, <name>!".
>
>
> *Use the `print()` function and proper string formatting.*
>
A sample solution might look like this:
def greet_user(name):
print(f"Hello, {name}!")
# Test your function
greet_user("CodenQuest")
Upon running the code, CodenQuest will check your output against the expected result, providing a quick pass/fail summary. If you pass, you move on; if not, you get hints or can analyze the test case outputs to see where you went wrong.
7. Tips for Mastering Python
While learning the basics is important, you’ll want to build good habits from day one to make your Python skills truly stand out.
- Practice Consistently: Make use of CodenQuest’s daily challenges. Even 15 minutes of consistent coding can lead to significant improvements.
- Explore Documentation: The official Python documentation is extremely comprehensive. Use it to look up details on functions, libraries, or data structures you’re unfamiliar with.
- Use External Resources: Supplement your learning with external tutorials and videos:
- Engage with the Community: Sites like Stack Overflow and Reddit’s r/learnpython are great places to ask questions and learn from others.
- Aim for Real Projects: After you’ve nailed the basics, try building a small web app, data analysis script, or automation script. Applying what you learn to real tasks will supercharge your skills.
- Upgrade to Premium (If it Makes Sense for You): For advanced guidance, CodenQuest Premium offers:
- Unlimited lives (no ad interruptions)
- Access to advanced challenges
- Custom editor themes for a more personalized coding environment
- An AI coding assistant that provides suggestions and debugging help
8. Conclusion
Learning Python can open doors to countless opportunities in software development, data science, automation, and more. The language’s simplicity makes it perfect for beginners, while its powerful libraries and frameworks cater to even the most advanced projects.
Key Takeaways
- Python Syntax: Focus on clean indentation and minimal use of semicolons or brackets.
- Variables & Data Types: Python’s dynamic typing system makes it flexible but be mindful of best practices to maintain clarity.
- Practice on CodenQuest: Leverage gamification and immediate feedback to accelerate your learning. With free lessons in Python, daily challenges, and an easy-to-use code editor, you’ll find learning enjoyable and convenient.
- Next Steps: Keep coding consistently, experiment with mini-projects, and explore the Python community to broaden your horizons.
Prompt for an Image: “Create a friendly illustration of a beginner Python programmer happily solving coding challenges on CodenQuest. The programmer is surrounded by floating Python symbols and icons representing variables, strings, and lists.”
If you’re ready to jump in, head over to CodenQuest’s Python Track, sign up, and start your coding journey today. Whether your goal is to learn from scratch, brush up on fundamentals, or practice for coding interviews, Python on CodenQuest is the perfect place to begin.
Additional Resources
- External Links:
Thank you for taking the time to read through this guide! Getting started with Python has never been easier, and with CodenQuest, you’ll have a supportive, interactive environment to help you reach your coding goals. Whether you’re aiming to break into tech, upgrade your skills, or teach a classroom of students, CodenQuest has you covered—from individual learning plans to classroom management tools for instructors and coding interview kits for recruiters.
Happy coding!