Understanding Variables in JavaScript: Declarations, Data Types, and Best Practices
Master the basics of variable declarations, scoping, and data types in JavaScript with this in-depth guide. Learn how to effectively use var, let, and const and gain a solid foundation for your coding journey.
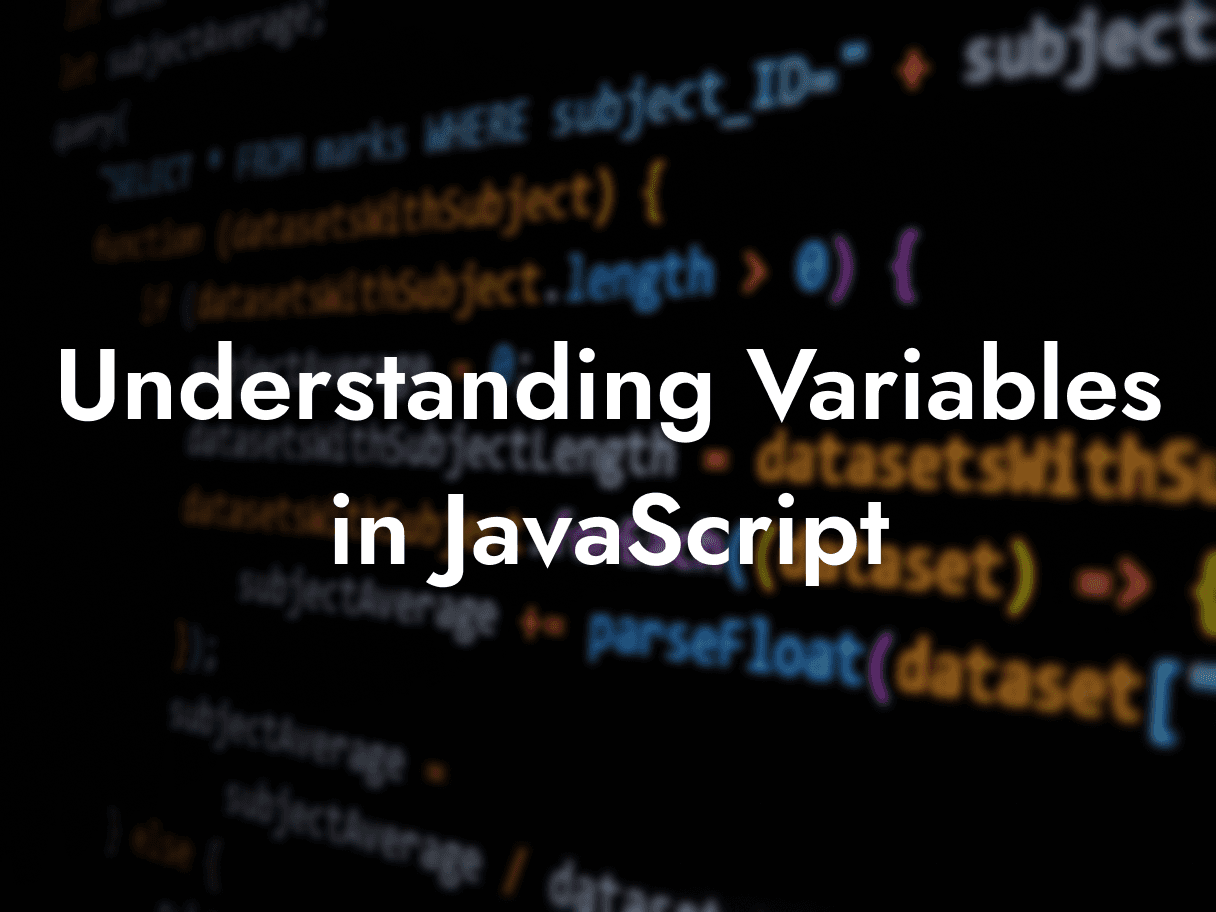
JavaScript is one of the most widely used programming languages today. Whether you’re building interactive websites, mobile apps, or server-side applications, you can’t escape variables—they’re everywhere in your code. Variables act as containers for storing values and are essential for creating dynamic functionality.
In this guide, we’ll explore everything you need to know about declaring variables in JavaScript, including an in-depth look at variable types, how to use them, and best practices. By the end of this post, you’ll have a solid foundation that will serve you well as you advance on your coding journey.
1. What Are Variables in JavaScript?
A variable in JavaScript is a named storage for data. Think of it like a box with a label—you can put different things inside, and whenever you want to use the contents, you just refer to the label. Variables make your code dynamic, allowing you to perform operations on stored data rather than using static values everywhere.
Example
let age = 25;
console.log(age); // Outputs: 25
age = 26;
console.log(age); // Outputs: 26
In this example, age
is a variable that initially stores the value 25
. We then update it to 26
.
2. Declaring Variables: var
, let
, and const
In modern JavaScript, there are three keywords you can use to declare variables:
var
(the old way, introduced in JavaScript from the start)let
(introduced in ES6/ES2015 for block-scoped variables)const
(introduced in ES6/ES2015 for block-scoped constants or read-only variables)
Let’s examine each one in detail.
2.1 Using var
(Older Way)
Historically, var
was the only way to declare a variable in JavaScript. However, it comes with a few quirks, particularly with respect to scoping.
var
variables are function-scoped, meaning they are visible throughout the entire function in which they’re declared.var
variables get hoisted, meaning they can be accessed before they’re declared (though their value isundefined
until the line of declaration is executed).
Example
function exampleVar() {
console.log(myVar); // undefined (due to hoisting)
var myVar = "Hello Var";
console.log(myVar); // "Hello Var"
}
Although myVar
is called before it’s declared, JavaScript doesn’t throw an error but returns undefined
. This can lead to confusing bugs, which is why modern code generally avoids var
.
2.2 Using let
(Block-Scoped)
let
was introduced in ES6 to fix some of the tricky issues with var
. The biggest difference is that let
is block-scoped, meaning it’s only accessible within the pair of curly braces ({}
) where it is declared. It also gets hoisted in a different manner, but you cannot use the variable before its declaration without causing an error.
Example
function exampleLet() {
// Uncomment the next line to see what happens
// console.log(myLetVar); // ReferenceError: Cannot access 'myLetVar' before initialization
let myLetVar = "Hello Let";
console.log(myLetVar); // "Hello Let"
}
if (true) {
let insideBlock = 10;
}
console.log(typeof insideBlock); // "undefined" because it’s out of the block scope
Because let
respects block scope, insideBlock
is not accessible outside the if
statement block.
2.3 Using const
(Immutable Bindings)
const
is very similar to let
in terms of block scope. However, the key difference is that variables declared with const
cannot be reassigned. Keep in mind that const
doesn’t make the data itself immutable if it’s an object or array; it only prevents you from reassigning the variable identifier to a new value.
Example
const myConstVar = "Hello Const";
console.log(myConstVar); // "Hello Const"
// This will throw an error
// myConstVar = "New Value"; // TypeError: Assignment to constant variable
// However, for objects:
const myConstObj = { prop: 1 };
myConstObj.prop = 2; // This is allowed because we're changing an internal value, not reassigning the entire object.
console.log(myConstObj.prop); // 2
3. JavaScript Data Types
JavaScript data types determine the kind of values you can store in variables. Understanding these types is crucial for writing bug-free and efficient code.
3.1 Number
JavaScript uses a single number type, which can represent both integers and floating-point values. You can perform various arithmetic operations on numbers.
let score = 10;
let price = 9.99;
let sum = score + price; // 19.99
3.2 String
A string in JavaScript is a sequence of characters. You can use single quotes, double quotes, or backticks (template literals) to declare a string.
let greeting = "Hello, world!";
let name = 'John Doe';
let welcomeMessage = `Hello, ${name}!`; // Template literal
3.3 Boolean
Boolean values are true
or false
. These are typically used in conditional statements and logic operations.
let isLoggedIn = true;
if (isLoggedIn) {
console.log("User is logged in");
} else {
console.log("User is not logged in");
}
3.4 Null
null
is an assignment value that explicitly indicates an empty or unknown value.
let data = null;
3.5 Undefined
undefined
means a variable has been declared but has not yet been assigned a value.
let result;
console.log(result); // undefined
3.6 Object
In JavaScript, an object is a collection of key-value pairs. You can think of it as a more flexible data structure.
const person = {
name: "Alice",
age: 30,
isStudent: false
};
console.log(person.name); // Alice
Arrays are a special kind of object in JavaScript, used to store ordered lists of values.
let numbers = [1, 2, 3];
console.log(numbers[0]); // 1
3.7 Symbol
Symbols are unique, immutable identifiers commonly used as keys for object properties to avoid name clashes.
let uniqueKey = Symbol("key");
let anotherKey = Symbol("key");
console.log(uniqueKey === anotherKey); // false
3.8 BigInt
BigInt is a numeric type that can represent integers in arbitrary precision, making it useful for working with very large numbers.
const bigNumber = 123456789012345678901234567890n;
console.log(bigNumber);
4. Variable Scope and Hoisting
4.1 Scope
Scope refers to where your variables and functions are accessible in your code. In JavaScript:
- Global scope: Variables declared outside any function or block are accessible everywhere.
- Function scope: Variables declared inside a function (
var
) are accessible only within that function. - Block scope: Variables declared with
let
orconst
inside any block ({ ... }
) are not accessible outside that block.
4.2 Hoisting
Hoisting is a mechanism where variable and function declarations move to the top of their scope before code execution. While both var
and function
declarations are hoisted, let
and const
declarations are somewhat “hoisted” but remain in a "temporal dead zone" until they’re actually declared.
console.log(hoistedVar); // undefined
var hoistedVar = "This is hoisted";
console.log(hoistedLet); // ReferenceError
let hoistedLet = "This is not accessible before declaration";
5. Best Practices
- Use
let
andconst
: Stick tolet
andconst
for variable declarations. Reserveconst
for variables that won’t be reassigned, and uselet
for reassignable variables. - Descriptive Names: Pick clear, concise, and meaningful names for variables. For example,
userName
ortotalPrice
. - Avoid Global Variables: Minimize the use of global variables to prevent name collisions and unexpected behavior.
- Initialize Variables: Always initialize variables with a value to avoid accidental
undefined
. - Use Strict Mode: Enable strict mode by adding
"use strict";
at the top of your file to catch common coding mistakes.
6. Further Resources
Below are some resources that can bolster your understanding of variables and JavaScript in general:
- JavaScript Array Questions for Beginners – a curated list of questions on CodenQuest to practice array operations.
- CodenQuest JavaScript Lessons
- Common JavaScript String Questions – question list focusing on string manipulations.
7. Conclusion
Understanding how to work with variables is vital in any programming language, and JavaScript is no exception. They’re the building blocks that let you store, manipulate, and manage data effectively. By mastering var
, let
, and const
, you’ll not only avoid common pitfalls like hoisting confusion but also write cleaner, more maintainable code.
Additionally, knowing the different data types—like Number, String, Boolean, Null, Undefined, Object, Symbol, and BigInt—will help you craft logical operations and data structures suited to your application’s needs. Remember to follow best practices such as using meaningful variable names, sticking with let
and const
, and avoiding globals whenever possible.
If you’re looking to further sharpen your skills, consider exploring the JavaScript Questions for Beginners on CodenQuest. You’ll learn how to manipulate arrays in various ways, which is a critical part of most JavaScript programs. For a more structured learning experience, check out our CodenQuest JavaScript Lessons where you can take bite-sized lessons, practice coding challenges, and track your progress.
Lastly, coding is best learned by doing—so fire up your editor or head over to CodenQuest to practice coding challenges in real-time. By consistently applying these fundamental concepts, you’ll be writing dynamic, bug-free, and organized JavaScript code in no time!