Top 10 Common Coding Interview Questions and How to Practice Them
Discover the top 10 coding interview questions, complete with example solutions and expert tips. Learn how to effectively practice them on CodenQuest to boost your coding confidence.
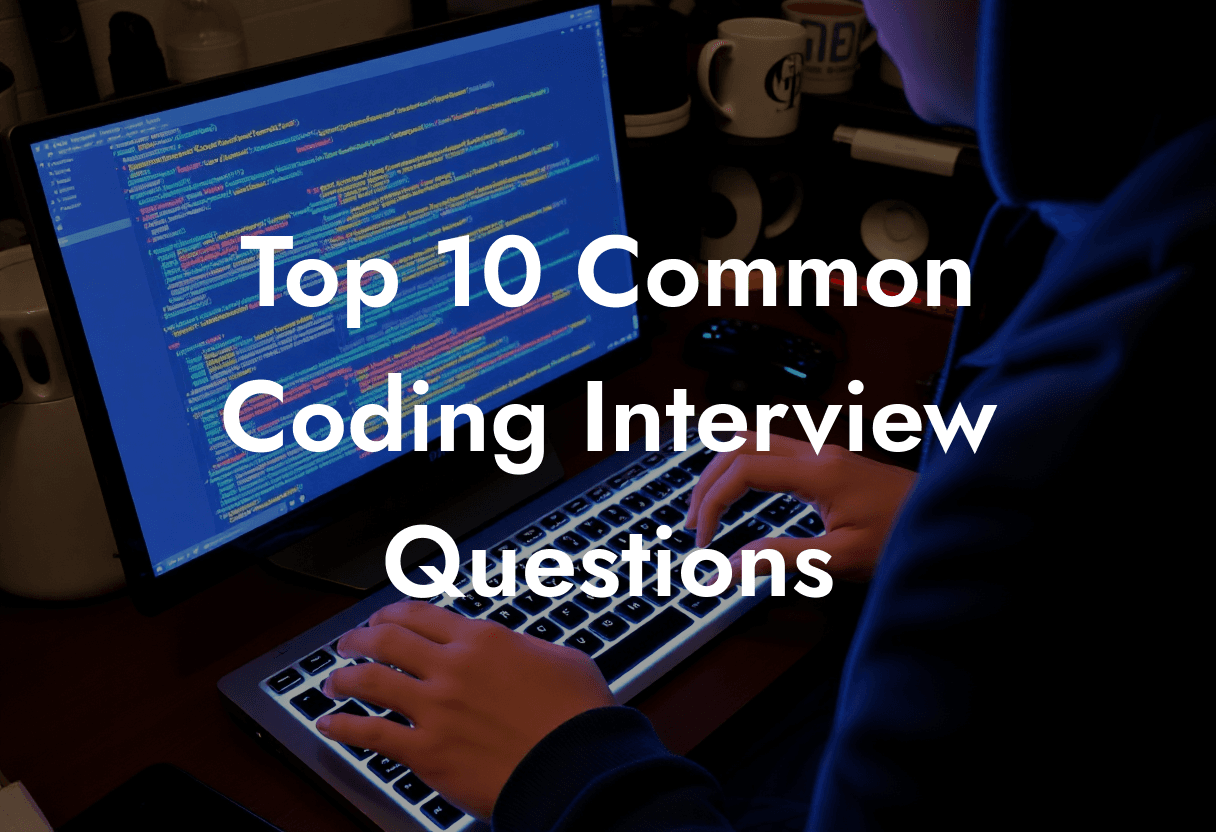
Whether you’re prepping for your first coding interview or you’re a seasoned developer looking to sharpen your skills, understanding classic interview questions is essential. These questions often revolve around fundamental data structures and algorithms, which companies use to gauge how you solve problems under pressure. In this article, we’ll explore 10 of the most common coding interview questions, provide clear solutions, and show you how to practice them using CodenQuest (an internal link you can add). Get ready to level up your skills and ace your next interview!
1. Two Sum
The Problem:
Given an array of integers and a target value, determine if any two numbers in the array add up to the target. Return their indices if they exist, or indicate that no such pair is found.
Why It’s Asked:
This is a classic test of your ability to use efficient lookups (like hash maps) and your familiarity with time complexity trade-offs. It also reveals how you handle edge cases.
Sample Solution in Python:
def two_sum(nums, target):
lookup = {}
for i, num in enumerate(nums):
complement = target - num
if complement in lookup:
return [lookup[complement], i] # return the pair of indices
lookup[num] = i
return None # or indicate no valid pair exists
# Example Usage:
nums = [2, 7, 11, 15]
target = 9
print(two_sum(nums, target)) # Output: [0, 1]
Practice directly on CodenQuest: https://codenquest.com/questions/two-sum
2. Reverse a Linked List
The Problem:
You’re given the head of a singly linked list. Reverse the list and return the new head.
Why It’s Asked:
Linked list manipulation is a popular interview topic because it checks how well you understand pointers and references. Reversing a linked list is also a building block for more complex linked list problems.
Sample Solution in Python:
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev, curr = None, head
while curr:
nxt = curr.next
curr.next = prev
prev = curr
curr = nxt
return prev
# Example Usage:
# Input: 1 -> 2 -> 3 -> None
# Output: 3 -> 2 -> 1 -> None
Practice directly on CodenQuest: https://codenquest.com/questions/reverse-linked-list
3. Valid Parentheses
The Problem:
Check if a given string containing only the characters (
, )
, {
, }
, [
, and ]
is valid. A valid string requires every opening bracket to have a matching closing bracket in the correct order.
Why It’s Asked:
This problem tests knowledge of stack operations and how well you can map pairs of characters.
Sample Solution in Python:
def is_valid_parentheses(s):
stack = []
mapping = {')': '(', '}': '{', ']': '['}
for char in s:
if char in mapping:
top_element = stack.pop() if stack else '#'
if mapping[char] != top_element:
return False
else:
stack.append(char)
return not stack
# Example Usage:
s = "()[]{}"
print(is_valid_parentheses(s)) # Output: True
Practice directly on CodenQuest: https://codenquest.com/questions/valid-parentheses
4. Merge Two Sorted Lists
The Problem:
Given two sorted linked lists, merge them into a single sorted list.
Why It’s Asked:
Combining two sorted data structures is a fundamental step in many algorithms (like merge sort). This question also tests your ability to traverse and manipulate linked lists.
Sample Solution in Python:
def merge_two_lists(l1, l2):
dummy = ListNode()
current = dummy
while l1 and l2:
if l1.val < l2.val:
current.next = l1
l1 = l1.next
else:
current.next = l2
l2 = l2.next
current = current.next
# Attach remaining elements
current.next = l1 if l1 else l2
return dummy.next
How to Practice on CodenQuest:
- Clone or fork a similar exercise from our question library.
- Use the hints feature if you get stuck or want to confirm your approach.
- Try a time-based challenge: set a 10-minute timer to replicate interview pressure.
Practice directly on CodenQuest: https://codenquest.com/questions/merge-two-sorted-lists
5. Maximum Subarray (Kadane’s Algorithm)
The Problem:
Find the contiguous subarray within a one-dimensional array of numbers that has the largest sum.
Why It’s Asked:
Kadane’s Algorithm is a well-known dynamic programming approach that interviews use to test your ability to optimize performance. A naive solution might have O(n^2) time complexity, while Kadane’s runs in O(n).
Sample Solution in Python:
def max_subarray(nums):
max_current = max_global = nums[0]
for i in range(1, len(nums)):
max_current = max(nums[i], max_current + nums[i])
if max_current > max_global:
max_global = max_current
return max_global
# Example Usage:
nums = [-2,1,-3,4,-1,2,1,-5,4]
print(max_subarray(nums)) # Output: 6 (subarray is [4, -1, 2, 1])
Practice directly on CodenQuest: https://codenquest.com/questions/maximum-subarray-sum
6. Binary Tree Inorder Traversal
The Problem:
Given the root of a binary tree, return its inorder traversal (left → node → right).
Why It’s Asked:
Tree traversal is a basic but crucial concept in computer science. It tests your understanding of recursion or stack usage in non-recursive approaches.
Sample Recursive Solution in Python:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
return inorder_helper(root, [])
def inorder_helper(node, result):
if not node:
return result
inorder_helper(node.left, result)
result.append(node.val)
inorder_helper(node.right, result)
return result
7. Climbing Stairs
The Problem:
You have to climb n
steps of stairs. You can either climb 1 or 2 steps at a time. How many distinct ways can you climb to the top?
Why It’s Asked:
This question tests fundamental dynamic programming concepts. It’s a friendly way to explore Fibonacci-like sequences, since the number of ways to climb n
stairs is the sum of ways to climb n-1
stairs and n-2
stairs.
Sample Solution in Python:
def climb_stairs(n):
if n <= 2:
return n
dp = [0]*(n+1)
dp[1], dp[2] = 1, 2
for i in range(3, n+1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Example Usage:
print(climb_stairs(5)) # Output: 8
Practice directly on CodenQuest: https://codenquest.com/questions/minimum-coins-for-toll
8. Merge Sort
The Problem:
Implement merge sort on an array of integers. Merge sort is a divide-and-conquer algorithm that recursively splits the array and then merges the sorted halves.
Why It’s Asked:
Sorting algorithms frequently appear in interviews. Merge sort demonstrates your knowledge of recursion, splitting arrays, and merging sorted subarrays. It’s also a solid introduction to algorithmic time complexity (O(n log n)).
Sample Solution in Python:
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr)//2
left = arr[:mid]
right = arr[mid:]
merge_sort(left)
merge_sort(right)
i = j = k = 0
# Merge the sorted halves
while i < len(left) and j < len(right):
if left[i] < right[j]:
arr[k] = left[i]
i += 1
else:
arr[k] = right[j]
j += 1
k += 1
# Copy the remaining elements
while i < len(left):
arr[k] = left[i]
i += 1
k += 1
while j < len(right):
arr[k] = right[j]
j += 1
k += 1
# Example Usage:
nums = [38, 27, 43, 3, 9, 82, 10]
merge_sort(nums)
print(nums) # Output: [3, 9, 10, 27, 38, 43, 82]
Practice directly on CodenQuest: https://codenquest.com/questions/merge-sort
9. Binary Search
The Problem:
Given a sorted array, find the position of a target value using binary search. If the target is not found, return -1 or an appropriate indicator.
Why It’s Asked:
Binary search is a fundamental algorithm that significantly reduces search time from O(n) to O(log n). Interviewers often expect you to understand it thoroughly, including edge cases.
Sample Solution in Python:
def binary_search(nums, target):
left, right = 0, len(nums) - 1
while left <= right:
mid = (left + right) // 2
if nums[mid] == target:
return mid
elif nums[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example Usage:
nums = [1, 3, 5, 7, 9, 11]
print(binary_search(nums, 7)) # Output: 3
Practice directly on CodenQuest: https://codenquest.com/questions/quicksort
10. Longest Common Prefix
The Problem:
Given an array of strings, find the longest common prefix (LCP) among them. If there is no common prefix, return an empty string.
Why It’s Asked:
String manipulation is a popular topic in interviews. The LCP problem tests your ability to compare strings efficiently and handle tricky edge cases (like empty strings or arrays).
Sample Solution in Python:
def longest_common_prefix(strs):
if not strs:
return ""
prefix = strs[0]
for s in strs[1:]:
while s[:len(prefix)] != prefix and prefix:
prefix = prefix[:len(prefix)-1]
if not prefix:
break
return prefix
# Example Usage:
strings = ["flower","flow","flight"]
print(longest_common_prefix(strings)) # Output: "fl"
Tips for Making the Most of Your Practice
- Track Your Progress: Use CodenQuest’s statistics feature to track how your solutions perform over time.
- Set Daily Goals: Consistency is key. Take advantage of our small daily challenges to ensure you’re always growing.
- Leverage Premium Features: Upgrading to premium unlocks unlimited problem access, AI coding assistance, and custom editor themes, all of which can help you polish your solutions faster.
- Explore Multi-Language Solutions: Interviewers may test you in a language you’re less familiar with. CodenQuest supports popular languages like Java, Python, JavaScript, C++, Go, and more.
Final Thoughts
Mastering these 10 common coding interview questions can significantly boost your confidence. Practice is the key ingredient—especially under realistic conditions where time is limited. Start by tackling these problems in your favorite language on CodenQuest, and watch your problem-solving skills grow day by day. Remember, the journey to becoming a standout candidate starts with consistent practice, staying curious, and never being afraid to explore new solutions.
Are you ready to take your coding interview skills to the next level? Sign up on CodenQuest or (https://codenquest.com) for iOS and Android, and begin your journey today!